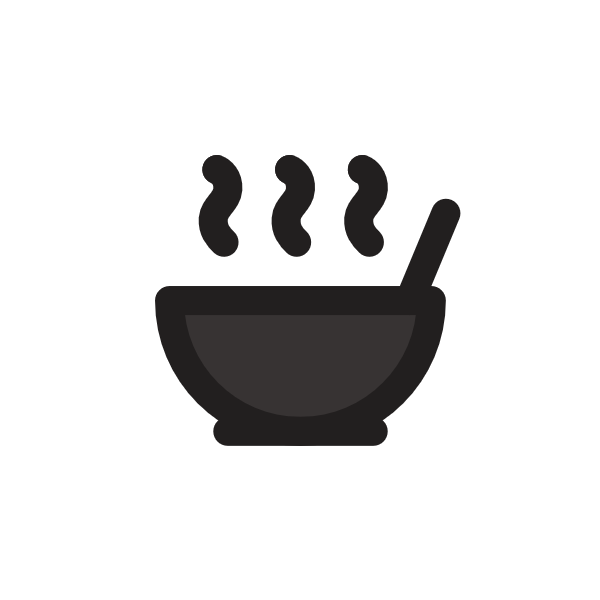
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Bit banging
Read the original article here.
The Forbidden Code: Underground Programming Techniques They Won’t Teach You in School
Module 3: Bypassing Hardware – The Art of Bit Banging
Welcome, initiates, to another session delving into the forbidden arts of programming. Today, we uncover a technique that bypasses conventional wisdom and dedicated hardware, forcing the humble CPU to perform tasks it wasn't inherently designed for in isolation. This technique is known as Bit Banging.
In standard academic settings, you're often taught to rely on dedicated hardware peripherals – specialized blocks designed to handle specific tasks like communication. They make life easy. But what happens when those peripherals aren't available? When you need to talk to a strange device with no standard interface? When every cent of hardware cost is critical? That's when you turn to bit banging.
What is Bit Banging?
Bit Banging: A technique for digital data transmission where software directly manipulates general-purpose input/output (GPIO) pins to send and receive data bits, simulating the function of dedicated communication hardware.
Essentially, instead of telling a dedicated chip "send this byte using I2C," you, the programmer, take manual control. You write code that says: "Set Pin 1 high, wait for 10 microseconds, set Pin 1 low, wait for 10 microseconds," and so on, meticulously replicating the required timing and logic of a communication protocol bit by bit.
The "Forbidden" Aspect
Why is this considered an "underground" or "forbidden" technique in some contexts?
- It's Resource Intensive: Bit banging consumes significant CPU time. The processor is tied up constantly monitoring or controlling a pin and managing precise timing, often preventing it from doing much else concurrently.
- It's Complex and Error-Prone: Getting the timing exactly right in software, especially on a system running other tasks or an operating system with unpredictable scheduling, is incredibly difficult. Small delays or interruptions can corrupt data.
- It Demands Deep Understanding: You need an intimate knowledge of the protocol you're implementing and the target hardware's timing characteristics and capabilities.
- It's Often a Last Resort: While powerful, it's typically used when dedicated hardware isn't an option because the standard, hardware-assisted methods are vastly more efficient and reliable.
It's the kind of technique you learn when facing real-world constraints that standard classroom examples don't cover – a true skill forged in the trenches of embedded systems development.
How Bit Banging Works: Manual Control
The core of bit banging lies in controlling GPIO pins.
General-Purpose Input/Output (GPIO): Digital pins on an integrated circuit (like a microcontroller or processor) that can be individually configured and controlled by software as either inputs (to read a voltage level, high or low) or outputs (to set a voltage level, high or low).
Imagine a GPIO pin as a simple switch you can flip on (set high, typically 3.3V or 5V) or off (set low, typically 0V) using software commands, and also check its current state (on or off).
To implement a communication protocol like, say, a basic serial transmit (like the transmit side of a UART), your software would perform these steps for each bit:
- Determine the value of the bit (0 or 1).
- Set the designated GPIO pin to the corresponding voltage level (low for 0, high for 1).
- Wait for the precise duration required by the protocol's baud rate.
- Repeat for the next bit.
Receiving data is similar but involves monitoring an input GPIO pin:
- Wait for a start condition (e.g., pin going low).
- Wait for a specific duration (often half the bit time) to sample the middle of the first data bit.
- Read the state of the GPIO pin (high or low) to determine the bit value.
- Wait for the full bit duration.
- Repeat for subsequent bits.
This meticulous timing is crucial. If your software is too slow or too fast, or gets interrupted, the receiving device won't correctly interpret the data stream.
Why Resort to Bit Banging? The Scenarios
Despite its drawbacks, bit banging is a vital tool in the underground programmer's arsenal. It's typically employed in situations where:
- Dedicated Hardware is Absent: The microcontroller or processor simply doesn't have a built-in peripheral for the required protocol (e.g., an older chip, a very low-cost chip, or a highly specialized custom interface).
- Cost is Paramount: Adding external hardware chips for communication (like a dedicated UART chip) costs money and board space. Bit banging uses existing GPIO pins, keeping hardware costs down.
- Flexibility and Custom Protocols: You need to implement a non-standard or proprietary communication protocol, or a standard protocol with unusual timing requirements, that dedicated hardware cannot handle.
- Low-Volume or Simple Tasks: For simple, low-speed communication that doesn't happen frequently, the overhead of bit banging might be acceptable compared to using or adding dedicated hardware.
- Hardware Bring-Up/Debugging: Sometimes, bit banging is used early in hardware development to test if a chip responds at all, before the complex drivers for dedicated peripherals are ready.
The Price of the Forbidden: Downsides and Challenges
Bit banging is powerful because it gives you direct control, but this control comes at a cost:
High CPU Load: As mentioned, the processor spends significant time executing the code that controls the GPIO pin and manages timing loops. This leaves less processing power for other tasks.
Timing Precision Issues: Software-based timing, especially on multitasking systems, is notoriously difficult to make perfectly consistent. Other processes, interrupts, or cache misses can introduce delays, leading to jitter.
Jitter: The deviation from true periodicity of a signal, often seen as variations in the timing between bits or pulses. In communication, excessive jitter can make data unreadable.
Signal Quality: The signals generated by bit banging can be less clean than those from dedicated hardware, potentially having glitches or slower rise/fall times depending on the GPIO pin's characteristics and the speed attempted.
Difficulty with High Speeds: Implementing high-speed protocols (like fast SPI or USB) via bit banging is often impossible due to the timing constraints and the processor's clock speed limitations relative to the protocol's requirements.
Complexity Scales with Protocol: Implementing simple protocols like one-wire interfaces or basic serial transmit is manageable. Implementing complex bidirectional protocols like I2C (which requires dynamic changing of pin direction and handling acknowledgements) or anything high-speed is significantly more challenging.
Bit Banging vs. Dedicated Hardware: The Core Trade-Off
This is the fundamental decision point:
- Dedicated Hardware (UART, SPI, I2C Peripherals):
- Pros: Handles protocol logic and timing automatically, significantly reduces CPU load (often just requiring software to load/read data registers), achieves higher speeds, provides better signal quality, often includes buffering.
- Cons: Requires the hardware peripheral to be present on the chip, less flexible for non-standard protocols, adds hardware cost/complexity if an external chip is needed.
- Bit Banging:
- Pros: Works with any available GPIO pin, allows implementation of any custom or standard protocol, zero additional hardware cost, ultimate flexibility.
- Cons: High CPU load, timing critical and prone to jitter, limited to lower speeds, requires complex software logic, poorer signal quality potentially.
The choice depends entirely on the constraints of your project: Do you have the hardware? Is cost critical? How fast does it need to be? How much CPU power can you spare?
Common Bit Banging Use Cases
Bit banging is frequently found in:
- Embedded Systems: The primary domain, where microcontrollers are resource-constrained and might lack specific peripherals.
- Interfacing Legacy/Unusual Devices: Communicating with old sensors, custom chips, or devices with non-standard serial interfaces.
- Simple Control Signals: Implementing basic protocols like Dallas 1-Wire temperature sensors, driving LED strips (like WS2812/NeoPixels which have precise timing requirements), or simple request/acknowledge lines.
- Driving Displays: Sometimes used for low-speed interfaces to simple LCDs or OLEDs.
- Bootstrapping/Initialisation: In some complex systems, a simple bit-banged interface might be used to configure a more complex chip before its dedicated hardware interface is active.
Conceptual Example: Bit-Banging a Simple Serial Transmit
Let's illustrate the concept without specific code, mimicking sending the byte 0x55
(binary 01010101
) using a hypothetical asynchronous serial protocol (like a simplified UART) at a "baud rate" requiring a 100 microsecond duration per bit.
Assume we have a GPIO pin configured as an output, currently held high (idle state).
- Start Bit: Set the GPIO pin low. Wait 100 microseconds. (Signals the start of a byte).
- Data Bit 0 (LSB first): The LSB of
01010101
is1
. Set the GPIO pin high. Wait 100 microseconds. - Data Bit 1: The next bit is
0
. Set the GPIO pin low. Wait 100 microseconds. - Data Bit 2: The next bit is
1
. Set the GPIO pin high. Wait 100 microseconds. - Data Bit 3: The next bit is
0
. Set the GPIO pin low. Wait 100 microseconds. - Data Bit 4: The next bit is
1
. Set the GPIO pin high. Wait 100 microseconds. - Data Bit 5: The next bit is
0
. Set the GPIO pin low. Wait 100 microseconds. - Data Bit 6: The next bit is
1
. Set the GPIO pin high. Wait 100 microseconds. - Data Bit 7 (MSB last): The MSB is
0
. Set the GPIO pin low. Wait 100 microseconds. - Stop Bit: Set the GPIO pin high. Wait at least 100 microseconds. (Signals the end of the byte, returns to idle state).
Every pin change and every delay must be precisely controlled by your software. If another process runs or an interrupt fires for too long during one of those 100-microsecond waits, the bit timing is off, and the receiving device might get corrupted data.
Advanced Considerations
For more reliable bit banging, especially when receiving data or dealing with faster speeds, techniques like:
Interrupt-Driven I/O: Configure the GPIO pin to trigger an interrupt when it changes state. The interrupt service routine (ISR) can then handle the precise timing and bit sampling/setting. This is significantly better than constant polling.
Polling: The process where software repeatedly checks the status of a device or input by reading a status register or pin state in a loop. It consumes CPU time continuously.
Interrupt: A signal to the processor from a hardware device or software event, indicating that a high-priority event has occurred and requesting the processor to suspend its current task to handle the event.
Optimized Assembly Code: For critical timing loops, writing the bit-banging code in assembly language can provide finer control over instruction timing.
Disabling Interrupts: Temporarily disabling interrupts during critical bit-banging sequences can prevent timing disruptions, but must be done cautiously as it can impact other parts of the system.
Conclusion
Bit banging is a testament to the power of software control over hardware. It's a technique born of necessity and constraint, allowing programmers to breathe life into basic pins and make devices communicate even when standard peripherals are unavailable. While often inefficient and challenging to implement perfectly, especially at higher speeds or on complex systems, it remains an indispensable "underground" skill in the world of embedded systems and low-level hardware interaction. Mastering it gives you the power to overcome hardware limitations and implement communication interfaces that the textbook solutions don't cover. It's not always the easiest path, but sometimes, it's the only path.